A Card Skeleton loading Animation using HTML and CSS
By Bytewebster - April 20, 2023
Welcome to bytewebster front-end projects. We're thrilled to have you here and excited to share our latest project with you. In this project, we'll be introducing you to our Card Skeleton loading Animation project - a front-end solution designed to enhance user experience.
Working
The Card skeleton loading animation is a popular UI design approach that shows users that the content is being loaded. It is frequently used in current web applications and mobile apps where enormous volumes of data must be retrieved from servers and the loading process can take several seconds.
Detailed Overview of Project
In this case, JavaScript is also used to implement the card skeleton loading animation, which is very useful when we need to load dynamic material or get data from an external source.
In this instance, JavaScript can be used to construct the skeleton animation, and AJAX or other data-fetching methods can be used to retrieve the actual data from the server. But since this is just a front-end project, for now we'll just tell you how to design it.
HTML Structure
We'll start by creating its HTML structure. This skeleton loading animation wasn't made using any framework. This has been created using purely HTML and CSS. Let's start making it.
First, it defines a container with two cards inside it. Each card has a title, some text, and two buttons. The first card is just a skeleton representation, while the second card contains actual content. Here's an explanation of the HTML code.
The first line starts with the container div tag with class "container". This defines a container to hold the cards inside it. Inside the container, there are two cards defined with the div tag and class "card".
The first card has three div tags inside it with class "card-img skeleton", "card-title skeleton", and "card-intro skeleton". These are just placeholder classes for styling purposes to create a skeleton representation of a card before the actual content is loaded.
<div class="container">
<div class="card">
<div class="card-img skeleton"></div>
<div class="card-body">
<h2 class="card-title skeleton"></h2>
<p class="card-intro skeleton"></p>
<div class="button-container">
<button class="card-btn skeleton"></button>
<button class="card-btn skeleton"></button>
</div>
</div>
</div>
<div class="card">
<div class="card-img">
<img src="" />
</div>
<div class="card-body">
<h2 class="card-title">
Civil War (2016)
</h2>
<p class="card-intro">
Lorem ipsum dolor sit amet consectetur adipisicing elit. Labore, veritatis!
</p>
<div class="button-container">
<button class="btn">Read More</button>
<button class="btn">Download</button>
</div>
</div>
</div>
</div>
And also the card has a button container with two buttons inside it, each with class "card-btn skeleton". These buttons also have the skeleton class to provide a placeholder for their visual representation.
The second card contains an image with the div tag and class "card-img". This is followed by a div tag with class "card-body" that contains the card's title and text.
Finally the card features a div with the class "button-container" that includes two buttons with the class "btn". These are fully functional buttons that users can interact with, unlike the buttons in the first card, which are simply placeholders. The buttons are labeled "Read More" and "Download" and provide users with a clear call to action, enabling them to explore further content or download relevant material.
Styling With CSS
As we mentioned earlier, we have used CSS to create this skeleton loading animation. So now we are moving on to the explanation of the CSS part of our project.
For small projects or simple animations like this card skeleton, it is common to use plain CSS. However, there are also other options available, such as SCSS, which can provide additional features and simplify the process of writing CSS, especially for larger projects.
The CSS defines several classes which are used to style the different components of the card, including the card container itself, the card image, the card title, and the card button. The .skeleton class is used to add the loading animation effect to the card.
The card is styled using flexbox, which allows for easy layout of the different components. The card container has a fixed width of 300px, and is not allowed to grow or shrink. It has a white background with a box shadow, and rounded corners.
.card {
display: flex;
flex-direction: column;
flex-basis: 300px;
flex-shrink: 0;
flex-grow: 0;
max-width: 100%;
background-color: #fff;
box-shadow: 0 5px 10px 0 rgba(0, 0, 0, 0.15);
border-radius: 10px;
overflow: hidden;
margin: 1rem;
}
.card-img {
padding-bottom: 56.25%;
position: relative;
}
.card-img img {
position: absolute;
width: 100%;
}
.card-body {
padding: 1.5rem;
}
.card-title {
font-size: 1.25rem;
line-height: 1.33;
font-weight: 700;
}
.card-title.skeleton {
min-height: 28px;
border-radius: 4px;
}
.card-intro {
margin-top: 0.75rem;
line-height: 1.5;
}
.card-intro.skeleton {
min-height: 72px;
border-radius: 4px;
}
.skeleton {
background-color: #e2e5e7;
background-image: linear-gradient(90deg, rgba(255, 255, 255, 0), rgba(255, 255, 255, 0.5), rgba(255, 255, 255, 0));
background-size: 40px 100%;
background-repeat: no-repeat;
background-position: left -40px top 0;
-webkit-animation: shine 1s ease infinite;
animation: shine 1s ease infinite;
}
@-webkit-keyframes shine {
to {
background-position: right -40px top 0;
}
}
@keyframes shine {
to {
background-position: right -40px top 0;
}
}
.container {
position: absolute;
top: 0;
left: 0;
right: 0;
bottom: 0;
width: 100%;
display: flex;
align-items: center;
justify-content: center;
flex-wrap: wrap;
}
.button-container {
display: flex;
justify-content: space-between;
align-items: center;
width: 100%;
margin-top: 1rem;
gap: 1rem;
}
.card-btn {
display: block;
width: 100%;
height: 44px;
border: none;
border-radius: 4px;
}
.card-btn.skeleton {
min-height: 44px;
}
.card:hover {
transform: translateY(-5px);
box-shadow: 0 10px 20px rgba(0, 0, 0, 0.15);
}
.card-img img {
transition: transform 0.5s ease;
}
.card:hover .card-img img {
transform: scale(1.1);
}
.btn {
display: block;
width: 100%;
height: 44px;
border: none;
border-radius: 4px;
background-image: radial-gradient(circle farthest-corner at 10% 20%, rgba(171, 102, 255, 1) 0%, rgba(116, 182, 247, 1) 90%);
font-size: 17px;
color: white;
cursor: pointer;
}
And the card image has a fixed aspect ratio of 16:9 and is positioned absolutely within its container. The card title and card intro have a minimum height and rounded corners to create the effect of a loading animation.
Next the .skeleton class is used to create a loading animation effect for the card. It sets a background color and adds a gradient effect to the background, which creates a shine effect as the animation progresses.
The animation property is used to create the animation effect, with the shine animation being defined in the @keyframes and @-webkit-keyframes rules.
Finally, the code defines a container to hold the card elements and a button container to hold the card button. The card has a hover effect, which makes the image scale up and the card lift up slightly. The button has a radial gradient background and changes color when hovered over.
Thank you for reading this article. We hope that you found the project engaging and informative.
Video of the Project
Take This Short Survey!
Download Source Code Files
From here You can download the source code files of this Card Skeleton loading Animation.
If you are new to web development, these code snippets can be helpful. If you find our blog posts valuable, we would be grateful if you could share them with others who are also interested in this topic.
ByteWebster Play and Win Offer.
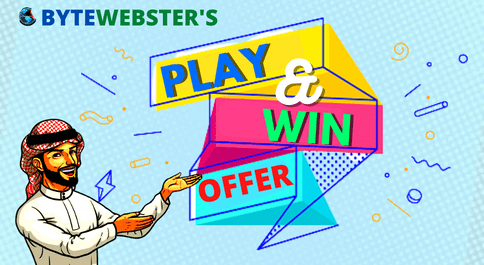
PLAY A SIMPLE GAME AND WIN PREMIUM WEB DESIGNS WORTH UPTO $100 FOR FREE.
PLAY FOR FREE