Build A Custom Captcha Using HTML, CSS, and JavaScript
By Bytewebster - April 02, 2023
Welcome to bytewebster javascript projects on building a custom captcha using HTML, CSS, and JavaScript.
In this project, we will tell you how you can easily create a custom captcha with the help of JavaScript.
You will also learn how to use JavaScript to create a unique captcha for your website.
This is a great way to improve your JavaScript skills.
Working
This JavaScript-made custom captcha is a way to add security to a website by providing a challenge that only humans can solve easily. It generates a random text using JavaScript which contains alphanumeric characters and displays it on an input field. The user has to enter the same text in a input box to verify they are human.
Detailed Overview of Project
As we all know that a captcha (Completely Automated Public Turing test to tell Computers and Humans Apart) is a method of determining whether a user is human or not by presenting a challenge that is simple for humans but difficult for bots. So there is no such thing in this that you do not know, still we are giving you its basic overview.
The most important thing is whether you use it or not. Even if you make it, you will learn something new in JavaScript and it may be useful to you sometime.
HTML Structure
Its HTML structure is also not very big so that it will be easy for you to understand the code. Perhaps we have made its interface quite complex. However, if you want, you can customize it according to your own need.
The code consists of a div element with a class of wrapper, which is the parent container for the whole interface. Inside the wrapper element, there is a header element that displays the text "JavaScript Captcha" along with an icon represented by an i element.
Below the header, there is a div element with a class of captcha-area, which contains the actual Captcha interface. The captcha-area is divided into two sections: The first section, which is a div element with a class of captcha-img, displays the Captcha image. The image itself is represented by a span element with a class of captcha.
<div class="wrapper">
<header><i class="bi bi-shield-lock"></i> JavaScript Captcha</header>
<div class="captcha-area">
<div class="captcha-img">
<span class="captcha"></span>
</div>
<button class="reload-btn"><i class="fas fa-redo-alt"></i> Refresh</button>
</div>
<form action="#" class="input-area">
<input type="text" placeholder="Enter Captcha" maxlength="6" spellcheck="false" required>
<button class="check-btn">Check</button>
</form>
<div class="status-text"></div>
</div>
<script src="script.js"></script>
The second section contains a button with a class of reload-btn. When clicked, this button refreshes the Captcha image. Below the captcha-area element, there is a form element with a class of input-area.
This form contains a single input element with a type attribute of text, a placeholder attribute with the text "Enter Captcha", a maxlength attribute of 6, a spellcheck attribute set to false, and the required attribute to ensure that the user enters a value before submitting the form.
Finally, there is a div element with a class of status-text. This element is initially empty, but it can be used to display a status message after the user submits the form.
Styling With CSS
This CSS code is used to style this captcha, The ".wrapper" class is used to set the maximum width, height, and background color of the form, as well as its border, padding, border-radius, and box-shadow. The header of the form is styled with the color, font-size, and font-weight attributes.
The ".captcha-area" class is used to display the captcha image and its reload button in a flexbox. The captcha image is styled with many CSS attributes.
Next the submit button is styled with the outline, border, color, cursor, background, border-radius, and transition attributes.
.wrapper{
max-width: 580px;
height: 290px;
width: 100%;
background: rgba( 255, 255, 255, 0.2 );
border: 1px solid rgba( 255, 255, 255, 0.18 );
padding: 22px 30px 40px;
border-radius: 10px;
box-shadow: rgba(0, 0, 0, 0.15) 2.4px 2.4px 3.2px;
}
.wrapper header{
color: white;
font-size: 30 px;
font-weight: 500;
}
.wrapper .captcha-area{
display: flex;
height: 65px;
margin: 30px 0 20px;
align-items: center;
justify-content: space-between;
}
.captcha-area .captcha-img{
height: 100%;
width: 405px;
user-select: none;
background: transparent;
border: 1px solid beige;
border-radius: 5px;
position: relative;
}
.captcha-img img{
width: 100%;
height: 100%;
object-fit: cover;
border-radius: 5px;
opacity: 0.95;
}
.captcha-img .captcha{
position: absolute;
left: 50%;
top: 50%;
width: 100%;
color: #fff;
font-size: 35px;
text-align: center;
letter-spacing: 10px;
transform: translate(-50%, -50%);
text-shadow: 0px 0px 2px #b1b1b1;
font-family: 'Noto Serif', serif;
}
.wrapper button{
outline: none;
border: none;
color: #fff;
cursor: pointer;
background: #5ce32a;
border-radius: 5px;
transition: all 0.3s ease;
border: 1px solid snow;
}
.wrapper button:hover{
background: #2fa5e9;
}
.captcha-area .reload-btn{
width: 160px;
height: 100%;
font-size: 25px;
margin: 5px;
}
.captcha-area .reload-btn i{
transition: transform 0.3s ease;
}
.captcha-area .reload-btn:hover i{
transform: rotate(15deg);
}
.wrapper .input-area{
height: 60px;
width: 100%;
position: relative;
}
.input-area input{
width: 100%;
height: 100%;
outline: none;
padding-left: 20px;
font-size: 20px;
border-radius: 5px;
border: 1px solid #bfbfbf;
}
.input-area input:is(:focus, :valid){
padding-left: 19px;
border: 2px solid #4db2ec;
}
.input-area input::placeholder{
color: #bfbfbf;
}
.input-area .check-btn{
position: absolute;
right: 7px;
top: 50%;
font-size: 17px;
height: 45px;
padding: 0 20px;
opacity: 0;
pointer-events: none;
transform: translateY(-50%);
}
.input-area input:valid + .check-btn{
opacity: 1;
pointer-events: auto;
}
Overall, this CSS code is used to create a visually appealing and functional captcha form that includes a captcha image, reload button, input field, and check button.
JavaScript Explanation
This JavaScript code is used to implement a custom CAPTCHA. Let's break down the code to understand it better.
The first line of code is used to select the HTML element with a class of "captcha" and assign it to the constant variable named "captcha". Similarly, the next four lines of code are used to select the HTML elements with classes of "reload-btn", "input-area input", "check-btn", and "status-text" and assign them to corresponding variables.
The next line declares an array called "allCharacters" containing all the possible characters that can be used to generate the CAPTCHA.
The "getCaptcha" function is used to generate the CAPTCHA. It uses a loop to generate six random characters from the "allCharacters" array and displays them in the "captcha" element using the "innerText" property.
const captcha = document.querySelector(".captcha"),
reloadBtn = document.querySelector(".reload-btn"),
inputField = document.querySelector(".input-area input"),
checkBtn = document.querySelector(".check-btn"),
statusTxt = document.querySelector(".status-text");
let allCharacters = ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O',
'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z', 'a', 'b', 'c', 'd',
'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's',
't', 'u', 'v', 'w', 'x', 'y', 'z', 0, 1, 2, 3, 4, 5, 6, 7, 8, 9];
function getCaptcha(){
for (let i = 0; i < 6; i++) {
let randomCharacter = allCharacters[Math.floor(Math.random() * allCharacters.length)];
captcha.innerText += ` ${randomCharacter}`;
}
}
getCaptcha();
reloadBtn.addEventListener("click", ()=>{
removeContent();
getCaptcha();
});
checkBtn.addEventListener("click", e =>{
e.preventDefault();
statusTxt.style.display = "block";
let inputVal = inputField.value.split('').join(' ');
if(inputVal == captcha.innerText){
Swal.fire({
icon: 'success',
title: 'Nice!',
text: 'You don\'t appear to be a robot.'
}).then(() => {
removeContent();
getCaptcha();
});
}else{
Swal.fire({
icon: 'error',
title: 'Oops...',
text: 'Captcha not matched. Please try again!'
});
}
});
function removeContent(){
inputField.value = "";
captcha.innerText = "";
statusTxt.style.display = "none";
}
The "getCaptcha" function is called once when the script is first loaded, to display the initial CAPTCHA. The "reloadBtn" event listener is used to call the "removeContent" function and then call the "getCaptcha" function again when the "reload" button is clicked.
The "checkBtn" event listener is used to check whether the user's input matches the generated CAPTCHA. It first gets the input value and replaces all spaces with an empty string, so that it can be compared with the generated CAPTCHA. If the input matches the CAPTCHA, a success message is displayed using the Swal (SweetAlert) library, and the CAPTCHA is regenerated. If the input does not match the CAPTCHA, an error message is displayed using the Swal library.
We appreciate you taking the time to read this article, and hope that you found the project to be enjoyable.
Video of the Project
Take This Short Survey!
Download Source Code Files
From here You can download the source code files of this JavaScript Custom Captcha.
If you are just starting in web development, these snippets will be useful. We would appreciate it if you would share our blog posts with other like-minded people.
ByteWebster Play and Win Offer.
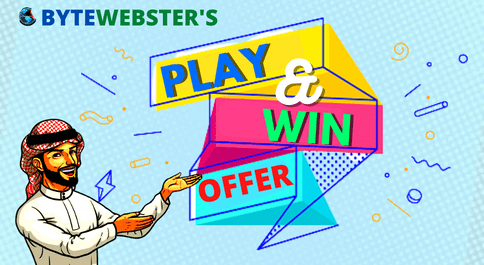
PLAY A SIMPLE GAME AND WIN PREMIUM WEB DESIGNS WORTH UPTO $100 FOR FREE.
PLAY FOR FREE