Learn How To Make A Weather Application Using weather API with HTML, CSS, and JavaScript
By Bytewebster - September 3, 2022
Welcome to the bytewebster Project blogs. Today we're going to create a new weather application with JavaScript and CSS.
We will not make this application without any major framework But to make this we will need a weather API We will also teach you how to use it in this project.
If you want to get success in JavaScript, then creating this weather app can prove to be useful for you.
Working:
To make this weather application, we first made its HTML and CSS structure Then to make it functional we used Weather API, and a lot of JavaScript's functions are also declared in it. inside the code of JavaScript, We will tell you the working and implementation of its API in the JavaScript part below.
Complete Detailed Overview of Project
To make this, you should know the basics of JavaScript and HTML for example JavaScript Objects, JavaScript HTML DOM, functions, If statements, ... etc. So let's see again how to make it.
HTML Structure
Its structure is very easy to make because in this you have to first create a search bar inside the div tag and a button with a search inside which you have to place the search icon.
After this, you have to make a card box in which you have to add things related to some weather application. For example, date, time, and location, and then make a row under the section of the unit with the temperature inside it.
There is not much major in this, but if you want to know a little more, then the code is given below, you can check all that directly.
<div class="container">
<div class="row">
<div class="col-sm-12 mx-auto">
<div class="card-box mx-auto">
<div class="search_icon">
<input type="text" class="search-bar" placeholder="Enter Location" id="searchTxt" />
<button class="button_icon" onclick="getdata()">
<i class="bi bi-search"></i>
</button>
</div>
</div>
<div class="card-box mx-auto">
<div class="weather">
<div class="city" id="location">Your Location</div>
<hr>
<div class="day" id="locationParts"><i class="bi bi-geo-alt"></i> Location</div>
<div class="day">
<p id="dateTime"><i class="bi bi-calendar"></i>  DateTime</p>
<p> | </p>
<p id="weekDay">Monday</p>
</div>
<div class="wheather">
<h1 class="temp" id="temperatureC">30°C</h1>
<h1> | </h1>
<h1 id="temperatureF">73°F</h1>
<img src="https://cdn.iconscout.com/icon/free/png-256/cloudy-weather-11-1147979.png" alt="" class="icon" id="weatherIcon" />
</div>
<div class="description" id="txtWord">Cloudy</div>
<div class="humidity" id="humidity">Humidity: 60%</div>
<div class="precipitation" id="precipitation">Precipitation: 4%</div>
<div class="wind" id="wind">Wind Speed: 6.2 km/h</div>
</div>
</div>
</div>
</div>
</div>
Styling With CSS
As you know any web applications and webpages look very bad without styling and like the rest of the projects, we will style this project as well.
We have styled some parts of it with the help of Bootstrap for which you have to paste the following CDN links in the head section of your HTML.
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-gH2yIJqKdNHPEq0n4Mqa/HGKIhSkIHeL5AyhkYV8i59U5AR6csBvApHHNl/vI1Bx" crossorigin="anonymous">
<!-- JavaScript Bundle with Popper -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0/dist/js/bootstrap.bundle.min.js" integrity="sha384-A3rJD856KowSb7dwlZdYEkO39Gagi7vIsF0jrRAoQmDKKtQBHUuLZ9AsSv4jD4Xa" crossorigin="anonymous"></script>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap-icons@1.9.1/font/bootstrap-icons.css">
When you will paste all the links there, then you have to add another link inside the head section which will be connected to the stylesheet (CSS) page.
While styling you only need to style the body tag, card box, input tags and some heading tags and try to get as much flex property as possible. because why flex properties are mostly responsive and mobile friendly.
@import url('https://fonts.googleapis.com/css2?family=Electrolize&display=swap');
*{
box-sizing: border-box;
margin: 0;
padding: 0;
}
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background: linear-gradient(to bottom, rgba(255,255,255,0.15) 0%, rgba(0,0,0,0.15) 100%), radial-gradient(at top center, rgba(255,255,255,0.40) 0%, rgba(0,0,0,0.40) 120%) #989898;
background-blend-mode: multiply,multiply;
font-family: "Open Sans", sans-serif;
}
/*styling card*/
.card-box {
background-image: linear-gradient(-20deg, #b721ff 0%, #21d4fd 100%);
color: white;
padding: 2em;
border-radius: 30px;
width: 600px;
margin: 1em;
box-shadow: rgb(38, 57, 77) 0px 20px 30px -10px;
}
.search_icon {
display: flex;
align-items: center;
justify-content: center;
}
.button_icon {
margin: 0.5em;
border: none;
height: 44px;
width: 52px;
font-size: 20px;
outline: none;
color: black;
background: linear-gradient(to bottom, #D5DEE7 0%, #E8EBF2 50%, #E2E7ED 100%), linear-gradient(to bottom, rgba(0,0,0,0.02) 50%, rgba(255,255,255,0.02) 61%, rgba(0,0,0,0.02) 73%), linear-gradient(33deg, rgba(255,255,255,0.20) 0%, rgba(0,0,0,0.20) 100%);
background-blend-mode: normal,color-burn;
cursor: pointer;
transition: 0.2s ease-in-out;
}
input.search-bar {
outline: none;
padding: 0.4em 1em;
color: black;
font-family: inherit;
font-size: 22px;
width: 520px;
height: 43px;
}
/*Styling placeholder*/
input.search-bar::placeholder {
color: gray;
}
button:hover {
background: black;
color: white;
}
.wheather {
width: 100vh;
flex-wrap: wrap;
display: flex;
flex-direction: row;
align-items: center;
}
.city {
font-size: 55px;
text-align: center;
font-family: 'Electrolize', sans-serif;
}
.wheather img {
margin-left: 130px;
height: 140px;
width: 170px;
}
.description {
font-size: 30px;
font-weight: bold;
}
.day {
display: flex;
font-size: 20px;
}
Script Overview
Now we will work on the most important part of it, which is its scripting because without JavaScript no web application can ever become fully functional.
You must be thinking that you will have to face the most trouble with the API while creating this but no, this is not such a big problem, you will be very soon familiar with it.
We have chosen a Weather API Provider to make this From there you can create weather apps in all types of programming languages like C, C++, Node.js, Java, Kotlin, and many more.
The API provider is www.rapidapi.com/
From here you will get the API syntax of each type of programming language already built, all you have to do is implement it in your code. and how to implement it, we have told you in the code given below, you must see all that.
async function getdata() {
var inputVal = document.getElementById("searchTxt").value;
const res = await fetch(
"https://weatherapi-com.p.rapidapi.com/current.json?q=q=" + inputVal, {
method: "GET",
headers: {
"x-rapidapi-host": "weatherapi-com.p.rapidapi.com",
"x-rapidapi-key": "4f8234a62amsh42185b0b78f249cp12e57ajsnb401d01fcbbf",
},
}
);
getWeekDay();
const data = await res.json();
document.getElementById("location").innerText = data.location.name;
document.getElementById("locationParts").innerHTML = "<i class='bi bi-geo-alt'></i> " +
data.location.region + " , " + data.location.country;
document.getElementById("dateTime").innerHTML = "<i class='bi bi-calendar'></i> " +
data.location.localtime.substr(0, 10);
document.getElementById("txtWord").innerText = data.current.condition.text;
document.getElementById("humidity").innerText =
"Humidity: " + data.current.humidity + "%";
document.getElementById("precipitation").innerText =
"Precipitation: " + data.current.precip_mm + "%";
document.getElementById("wind").innerText =
"Wind: " + data.current.wind_kph + "km/h";
document.getElementById("temperatureC").innerText =
data.current.temp_c + " °C";
document.getElementById("temperatureF").innerText =
data.current.temp_f + " °F";
document.getElementById("weatherIcon").src =
"https:" + data.current.condition.icon;
}
function getWeekDay() {
const weekday = [
"Sunday",
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday",
];
const d = new Date();
let day = weekday[d.getDay()];
document.getElementById("weekDay").innerText = day;
}
Video of the Project
Take This Short Survey!
JavaScript Weather App [Source Code Files]
From here You can download the source code files of this JavaScript Weather App If you are just starting out in web development, this snippets will be useful. We would appreciate it if you would share our blog posts with other like-minded people.
ByteWebster Play and Win Offer.
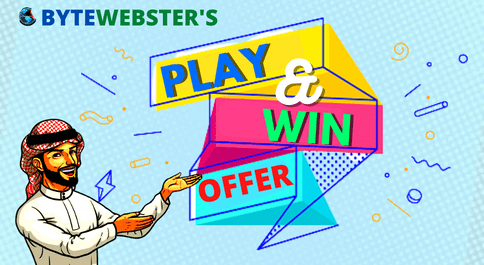
PLAY A SIMPLE GAME AND WIN PREMIUM WEB DESIGNS WORTH UPTO $100 FOR FREE.
PLAY FOR FREE