Create A Contact Form in Laravel 9 and store the Data in MySQL database
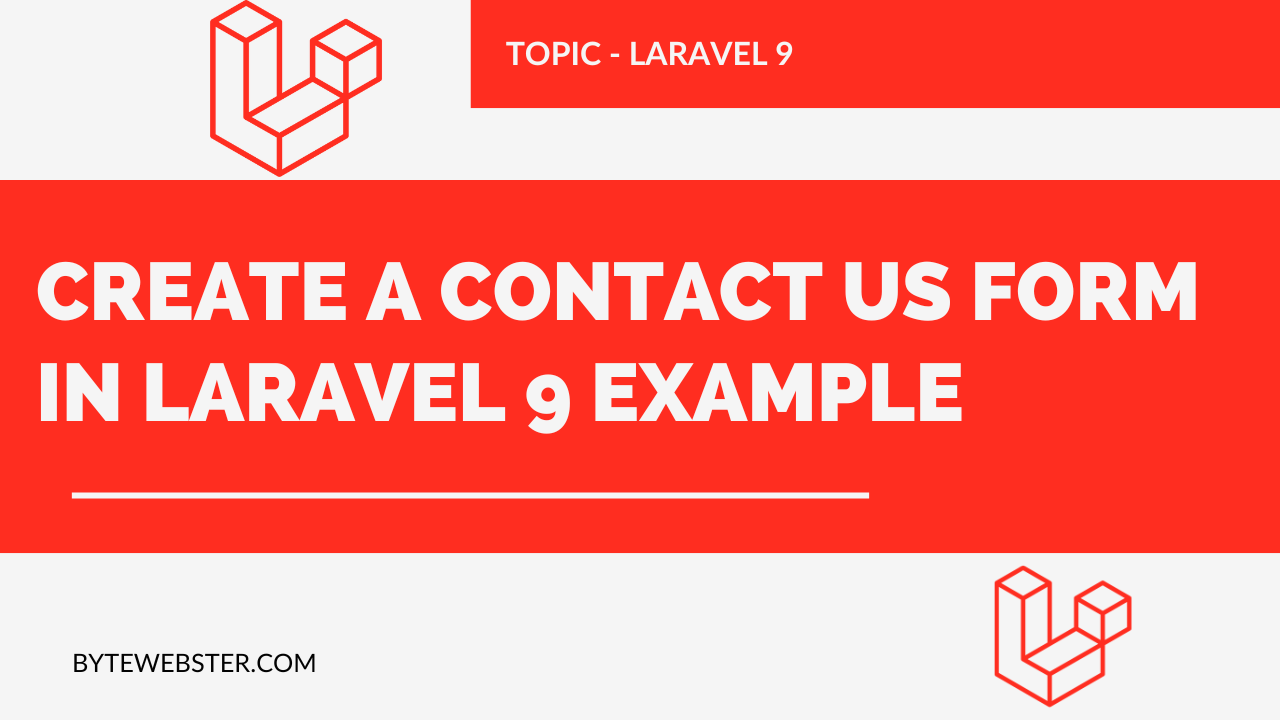
In this tutorial, we will create a contact us form in Laravel 9 and we will store the data of the form in MYSQL database. and after that we will also fetch or retrieve the data from the form database.
We have used Bootstrap Framework to design the structure of this form, you can take any other framework if you want or you can style it by writing your own CSS.
First of all, create a project of Laravel with this command.
composer create-project laravel/laravel contact-form
Give Database Connection
After this, you go directly to the .env file and give the information and details of your database. By doing this your Laravel project will be connected to your MySQL database.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=lara
DB_USERNAME=root
DB_PASSWORD=
Make Model & Run Database Migrations
Now that we have given the database connection, now we will create the model and run the migration A model and a migration file will be created automatically in your laravel project by running the following command.
php artisan make:model Contact -m
then we have to setup the structure of the table, for this we have to specify the values in the migration file. And with this we also need to declare those values in the model Contact.php
Now from here our real work begins find this create_contact_table.php file in the migration folder inside your database folder and put the below code in this page.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('contacts', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email');
$table->string('subject');
$table->text('message');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('contact');
}
};
Till now the structure of the table has been made, and now we have to prepare the model of this form also. For this you just have to put the following code in the file of your created model app/Models/Contact.php
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Contact extends Model
{
use HasFactory;
protected $fillable = [
'name',
'email',
'subject',
'message'
];
}
After this, the migration command has to be run. it will publish all our schema to the database. This command also creates the table in the database.
php artisan migrate
Make A Controller
Create a controller for your form using this given command
php artisan make:controller ContactController
Open the controller ContactController.php which you have created and enter the following code in it
<?php
namespace App\Http\Controllers;
use App\Models\Contact;
use Illuminate\Http\Request;
class ContactController extends Controller
{
public function contact_us(Request $request){
$this->validate($request, [
'name' => 'required',
'email' => 'required|email',
'subject'=>'required',
'message' => 'required'
]);
Contact::create($request->all());
return redirect('contact')->with('message', 'Thanks for contacting us! We will be in touch with you shortly.');
}
public function get_messages(){
$contacts=Contact::orderBy('id','desc')->paginate(10);
return view("get_messages",['contacts'=>$contacts]);
}
public function delete_message($id){
Contact::destroy($id);
// echo "deleted";
return redirect('get_messages')->with('message', 'Message Deleted Successfully');
}
}
Inside this controller, we have created three functions. In which the first function is to store the user's data in the database. And another function is to display all the contacts in the form of a table on a separate page And the last one which is our function is to delete those contacts.
And if you delete any contact then at the same time you will see a flash message that will be written Message Deleted Successfully.
Define Routes
Under Routes folder open the web.php file here we will define our routes.
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\ContactController;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/contact', function () {
return view('contact');
})->name("contact");
Route::post('/contact_us', [ContactController::class, 'contact_us'])->name("contact_us");
Route::get('/get_messages', [ContactController::class, 'get_messages'] )
->name("get_messages");
The first route we have created in this is only to show the contact form, that's why it is created with the GET method.
Our second route is made for the post method. This route works a lot like validating the form, Simultaneously deleting contacts messages, and inserting the data of the form into the database.
And also to show a success message as soon as you submit the form.
The last route is also created with the GET method, in this route, all the contact messages will be visible. And with every single message, there will also be an option to delete it.
Create Contact Us Form
The work is not completed yet now you have to create a laravel contact us form file contact.blade.php in resources/views/ folder
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Laravel Contact Us Form - ByteWebster</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-Zenh87qX5JnK2Jl0vWa8Ck2rdkQ2Bzep5IDxbcnCeuOxjzrPF/et3URy9Bv1WTRi" crossorigin="anonymous">
</head>
<body>
<h3 class="text-center">Contact Us - Project</h3>
<div class="col-sm-4 mx-auto">
<div class="container">
<div class="row justify-content-center">
<form action="{{ route('contact_us')}}" method="post" class="border p-5 rounded-2">
@csrf
@if(session('message'))
<div class="alert alert-success text-center">
{{ session('message') }}
</div>
@endif
<!-- name -->
<div class="mb-3">
<label class="form-label" for="name">Name</label>
<input type="text" name="name" placeholder="Enter Name" value="{{ old('name')}}" class="form-control">
@error('name')
<span class="text-danger">{{ $message }}</span>
@enderror
</div>
<!-- email -->
<div class="mb-3">
<label class="form-label" for="email">E-mail</label>
<input type="text" value="{{ old('email')}}" name="email" placeholder="Enter E-mail" class="form-control">
@error('email')
<span class="text-danger">{{ $message }}</span>
@enderror
</div>
<!-- subject -->
<div class="mb-3">
<label class="form-label" for="subject">Subject</label>
<input type="text" value="{{ old('subject')}}" name="subject" placeholder="Enter Subject" class="form-control">
@error('subject')
<span class="text-danger">{{ $message }}</span>
@enderror
</div>
<!-- message -->
<div class="mb-3">
<label class="form-label" for="message">Message</label>
<textarea class="form-control" value="{{ old('message')}}" name="message" placeholder="Enter Message"></textarea>
@error('message')
<span class="text-danger">{{ $message }}</span>
@enderror
</div>
<!-- submit -->
<div class="text-center">
<input type="submit" name="submit" value="submit" class="btn btn-primary">
</div>
</form>
</div>
</div>
</div>
</body>
</html>
Show All The Contacts Messages
By the way, till now the laravel contact form is completely ready but we want that we do not have to open the database again and again to see all the contacts. That's why we will show all those contact messages on a separate page. For this we have to fetch the data from our project database.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Laravel 9 Contact Us Form All Messages - Bytewebster</title>
</head>
<body>
<h1 class="text-center">Contact Form Messages</h1>
<div class="col-sm-12 mx-auto">
<div class="container">
<div class="row">
<div class="mx-auto">
@if(session('message'))
<div class="alert alert-warning fs-5">
{{ session('message')}}
</div>
@endif
</div>
<input type="text" id="myInput" onkeyup="myFunction()" placeholder="Search for messages..">
<div class="table-responsive">
<table class="table table-dark table-striped table-hover border rounded" id="myTable">
<thead>
<tr>
<th scope="col"><h3>Name</h3></th>
<th scope="col"><h3>E-Mail</h3></th>
<th scope="col"><h3>Subject</h3></th>
<th scope="col"><h3>Message</h3></th>
<th scope="col" class="text-center"><h3>Delete</h3></th>
</tr>
</thead>
<tbody>
@foreach($contacts as $contact)
<tr>
<td>{{ $contact['name']}}</td>
<td>{{ $contact['email']}}</td>
<td>{{ $contact['subject']}}</td>
<td>{{ $contact['message']}}</td>
<td class="text-center">
<a
href="{{ route('delete_message', ['id'=>$contact['id']])}}"
class="btn btn-danger btn-sm mb-1"><i class="bi bi-trash3-fill"></i></a>
</td>
</tr>
@endforeach
</tbody>
</table>
</div>
<div class="text-center">{{$contacts->links("pagination::bootstrap-5")}}</div>
</div>
</div>
</div>
</body>
</html>
Now our Laravel 9 made contact us form is completely ready.