Make A Responsive Carousel Using HTML, CSS Flexbox, and SwiperJS
By Bytewebster - May 25, 2023
Welcome to Bytewebster! In this JavaScript project, we will tell you about the use of SwiperJS.
We have also created a responsive carousel using HTML, CSS Flexbox, and SwiperJS.
So let's know about how it works and what is its use.
Working
If you don't know what SwiperJS is, let us explain before moving forward with this project. SwiperJS is a powerful JavaScript library that is commonly used for creating sliders or carousels in web projects. In this project, we have also created an image carousel slider that consists of 5 cards. Each card contains written details and also includes 2 additional small fields.
Detailed Overview of Project
Most of you may already know what carousels are used for. If you do not know, then let us tell you that a carousel is a very common component in web designing. Many front-end developers use carousels to make websites more attractive.
We also have other types of carousels in our projects section that you might find useful. Like here, we have an image carousel slider that is made of normal Javascript. And this is the second one, which is the Product Card Slider, which is the same as a carousel but with some differences.
HTML Structure
Now let's talk about its HTML structure, to build this responsive carousel, we will create a basic HTML structure to form the base of this swiperJS carousel.
The HTML code starts with a section element. Here we use the section element to cover the entire carousel because It allows for easier styling and targeting of the carousel section using CSS or JavaScript.
Then, inside the section, there is a div element with a class of "swiper". We used this because it acts as a wrapper that holds all the individual slides. The "swiper" class also plays a important role in integrating and interacting with the SwiperJS library.
<section>
<div class="swiper">
<div class="swiper-wrapper">
<div class="swiper-slide swiper-slide--one">
<span>domestic</span>
<div>
<h2>Enjoy the exotic of sunny Hawaii</h2>
<p>
<svg xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 24 24" stroke-width="1.5" stroke="currentColor" class="w-6 h-6">
<path stroke-linecap="round" stroke-linejoin="round" d="M15 10.5a3 3 0 11-6 0 3 3 0 016 0z" />
<path stroke-linecap="round" stroke-linejoin="round" d="M19.5 10.5c0 7.142-7.5 11.25-7.5 11.25S4.5 17.642 4.5 10.5a7.5 7.5 0 1115 0z" />
</svg>
Maui, Hawaii
</p>
</div>
</div>
<div class="swiper-slide swiper-slide--two">
<span>subtropical</span>
<div>
<h2>The Island of Eternal Spring</h2>
<p>
<svg xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 24 24" stroke-width="1.5" stroke="currentColor" class="w-6 h-6">
<path stroke-linecap="round" stroke-linejoin="round" d="M15 10.5a3 3 0 11-6 0 3 3 0 016 0z" />
<path stroke-linecap="round" stroke-linejoin="round" d="M19.5 10.5c0 7.142-7.5 11.25-7.5 11.25S4.5 17.642 4.5 10.5a7.5 7.5 0 1115 0z" />
</svg>
Lanzarote, Spanien
</p>
</div>
</div>
<div class="swiper-slide swiper-slide--three">
<span>history</span>
<div>
<h2>Awesome Eiffel Tower</h2>
<p>
<svg xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 24 24" stroke-width="1.5" stroke="currentColor" class="w-6 h-6">
<path stroke-linecap="round" stroke-linejoin="round" d="M15 10.5a3 3 0 11-6 0 3 3 0 016 0z" />
<path stroke-linecap="round" stroke-linejoin="round" d="M19.5 10.5c0 7.142-7.5 11.25-7.5 11.25S4.5 17.642 4.5 10.5a7.5 7.5 0 1115 0z" />
</svg>
Paris, France
</p>
</div>
</div>
<div class="swiper-slide swiper-slide--four">
<span>Mayans</span>
<div>
<h2>One of the safest states in Mexico</h2>
<p>
<svg xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 24 24" stroke-width="1.5" stroke="currentColor" class="w-6 h-6">
<path stroke-linecap="round" stroke-linejoin="round" d="M15 10.5a3 3 0 11-6 0 3 3 0 016 0z" />
<path stroke-linecap="round" stroke-linejoin="round" d="M19.5 10.5c0 7.142-7.5 11.25-7.5 11.25S4.5 17.642 4.5 10.5a7.5 7.5 0 1115 0z" />
</svg>
The Yucatan, Mexico
</p>
</div>
</div>
<div class="swiper-slide swiper-slide--five">
<span>native</span>
<div>
<h2>The most popular yachting destination</h2>
<p>
<svg xmlns="http://www.w3.org/2000/svg" fill="none" viewBox="0 0 24 24" stroke-width="1.5" stroke="currentColor" class="w-6 h-6">
<path stroke-linecap="round" stroke-linejoin="round" d="M15 10.5a3 3 0 11-6 0 3 3 0 016 0z" />
<path stroke-linecap="round" stroke-linejoin="round" d="M19.5 10.5c0 7.142-7.5 11.25-7.5 11.25S4.5 17.642 4.5 10.5a7.5 7.5 0 1115 0z" />
</svg>
Whitsunday Islands, Australia
</p>
</div>
</div>
</div>
<div class="swiper-pagination"></div>
</div>
</section>
Each carousel slide is represented by a div element with a specific class, and it contains the content for that particular slide. After that the slide also consists of a span element along with an SVG icon.
The SVG icon is specified using the svg element and includes path data for the icon design. The icon is displayed alongside the corresponding location text.
Finally, at the end of the "swiper" class container, there is a div element with a class of "swiper-pagination". It is used to display pagination bullets for navigation between the carousel slides. Now let's go to the designing part of this responsive carousel.
Styling With CSS
Now we will discuss the styling of this carousel. In this, we will learn from each class in detail how this carousel is made. Let's go through each section of the CSS code to understand how its works.
Firstly, the code starts with the section class. The section selector styles the container element for the carousel. It sets the position to relative It also uses a flexbox to centre the carousel vertically and horizontally within the section.
After that, the swiper class is applied to the carousel container element. It is applied because it sets the width to 100% to ensure the carousel occupies the full width of its parent container. then comes the swiper-slide class, which represents each slide within the carousel. The class sets a fixed width and height for each slide.
section {
position: relative;
width: 100%;
min-height: 100vh;
display: flex;
justify-content: center;
align-items: center;
background-image: radial-gradient( circle farthest-corner at 10% 20%, rgba(171,102,255,1) 0%, rgba(116,182,247,1) 90% );
overflow: hidden;
}
.swiper {
width: 100%;
padding-top: 50px;
padding-bottom: 50px;
}
.swiper-slide {
width: 300px;
height: 400px;
box-shadow: 0 15px 50px rgba(0, 0, 0, 0.2);
filter: blur(1px);
border-radius: 10px;
display: flex;
flex-direction: column;
justify-content: end;
align-items: self-start;
}
.swiper-slide-active {
filter: blur(0px);
}
.swiper-pagination-bullet,
.swiper-pagination-bullet-active {
background: #fff;
}
.swiper-slide span {
text-transform: uppercase;
color: #fff;
background: #1b7402;
padding: 7px 18px 7px 25px;
display: inline-block;
border-radius: 0 20px 20px 0px;
letter-spacing: 2px;
font-size: 0.8rem;
font-family: "Open Sans", sans-serif;
}
.swiper-slide--one span {
background: #62667f;
}
.swiper-slide--two span {
background: #087ac4;
}
.swiper-slide--three span {
background: #b45205;
}
.swiper-slide--four span {
background: #087ac4;
}
.swiper-slide h2 {
color: #fff;
font-family: "Roboto", sans-serif;
font-weight: 400;
font-size: 1.3rem;
line-height: 1.4;
margin-bottom: 15px;
padding: 25px 45px 0 25px;
}
.swiper-slide p {
color: #fff;
font-family: "Roboto", sans-serif;
font-weight: 300;
display: flex;
align-items: center;
padding: 0 25px 35px 25px;
}
.swiper-slide svg {
color: #fff;
width: 22px;
height: 22px;
margin-right: 7px;
}
.swiper-slide--one {
background: linear-gradient(to top, #0f2027, #203a4300, #2c536400),
url(https://images.unsplash.com/photo-1556206079-747a7a424d3d?ixlib=rb-4.0.3&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=871&q=80)
no-repeat 50% 50% / cover;
}
.swiper-slide--two {
background: linear-gradient(to top, #0f2027, #203a4300, #2c536400),
url("https://images.unsplash.com/photo-1571900670723-a317a66e3fb7?ixlib=rb-4.0.3&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=423&q=80")
no-repeat 50% 50% / cover;
}
.swiper-slide--three {
background: linear-gradient(to top, #0f2027, #203a4300, #2c536400),
url("https://images.unsplash.com/photo-1549144511-f099e773c147?ixlib=rb-4.0.3&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=387&q=80")
no-repeat 50% 50% / cover;
}
.swiper-slide--four {
background: linear-gradient(to top, #0f2027, #203a4300, #2c536400),
url("https://images.unsplash.com/photo-1650367310179-e1b5b8e453c3?ixlib=rb-4.0.3&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=870&q=80")
no-repeat 50% 50% / cover;
}
.swiper-slide--five {
background: linear-gradient(to top, #0f2027, #203a4300, #2c536400),
url("https://images.unsplash.com/photo-1596521864207-13d46b1a0c78?ixlib=rb-4.0.3&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=774&q=80")
no-repeat 50% 50% / cover;
}
.swiper-3d .swiper-slide-shadow-left,
.swiper-3d .swiper-slide-shadow-right {
background-image: none;
}
Next, the swiper-slide-active class is applied to the currently active slide. It removes the blur effect by setting the filter property. And after that the swiper-pagination-bullet and swiper-pagination-bullet-active classes style the pagination bullets for the carousel.
Finally, in the CSS code of this carousel, the swiper-slide span selector styles the text element within each slide. It sets the text to uppercase using the text-transform property. Mainly The purpose of this CSS code is to enhance the visual presentation of the carousel and its individual slides.
JavaScript Explanation
Now coming to the most important part, due to which this carousel has become possible, which is the swiper JS library, And here also, we have written the code for this library only. Because its code is not very long, you will not have much difficulty understanding it. So let's break down this Javascript code.
The JavaScript code of this carousel starts by initialising a new instance of Swiper and selecting the HTML element with the class name "swiper" to apply the carousel. Then there is an effect: "coverflow." This effect creates a 3D-like coverflow animation when transitioning between slides.
var swiper = new Swiper(".swiper", {
effect: "coverflow",
grabCursor: true,
centeredSlides: true,
slidesPerView: "auto",
coverflowEffect: {
rotate: 0,
stretch: 0,
depth: 100,
modifier: 2,
slideShadows: true
},
spaceBetween: 60,
loop: true,
pagination: {
el: ".swiper-pagination",
clickable: true
}
});
Then grabCursor: true changes the cursor to a grab cursor when hovering over the carousel; after that, there is centeredSlides: true. which ensures that the active slide is always centred in the carousel view.
For navigation, we have given pagination bullets so that it is easy to navigate the carousel. Means that it configures the pagination for the carousel. It specifies the HTML element with the class name "swiper-pagination" as the pagination container. Finally, there is the clickable: true option, which allows the pagination bullets to be clickable.
We are grateful for your time and attention, and we trust that you have found the project to be interesting.
Video of the Project
Take This Short Survey!
Download Source Code Files
From here You can download the source code files of this Responsive SwiperJS Carousel.
If you are just starting in web development, these snippets will be useful. We would appreciate it if you would share our blog posts with other like-minded people.
ByteWebster Play and Win Offer.
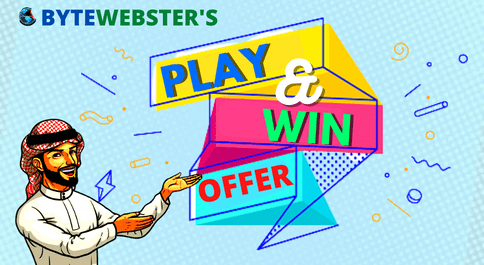
PLAY A SIMPLE GAME AND WIN PREMIUM WEB DESIGNS WORTH UPTO $100 FOR FREE.
PLAY FOR FREE